Design Patterns are used to write better maintainable and more efficient code. We usually write code to solve problems, and when those problems arise, we can notice that some of them are similar: they have common patterns. Which is where Design Patterns come: they are solutions to those common problems, and since they’ve been tried and tested by pros, you know you can trust them.
They’re very handy as they make code more reusable and readable, which saves a great deal of time in the development process (unless you wrongly identify the problem and try to apply a wrong pattern… in which case, it’ll be a bit of a disaster!).
There are a lot of different Design Patterns, which can be classified in several categories:
- Creational, which solves a problem by controlling the creation process of an object. Examples are: Constructor Pattern, Factory Pattern, Prototype Pattern, Singleton Pattern.
- Structural, which deals with object relationships, ensuring that if one part of a system changes, the entire system doesn’t need to change along with it. Examples are: Adapter Pattern, Composite Pattern, Decorator Pattern, Facade Pattern, Flyweight Pattern, Proxy Pattern.
- Behavioural, which improves communication between dissimilar objects. Examples are: Chain of Responsibility Pattern, Command Pattern, Iterator Pattern, Mediator Pattern, Observer Pattern, State Pattern, Strategy Pattern, Template Pattern.
In this article, we will only discuss one of them, the Factory Pattern, but I will add links at the bottom if you’re interested in the other ones too.
THE FACTORY PATTERN
A factory function is any function which is not a class or constructor that returns a (presumably new) object. In JavaScript, any function can return an object. When it does so without the “new” keyword, it’s a factory function.
They’re handy as they save you a lot of headaches, not having to deal with complex classes, the “new” and the “this” keywords, which are both nightmares (to me anyway).
The Factory pattern can be especially useful when applied to the following situations:
- When the object or component setup involves a high level of complexity.
- When we need to easily generate different instances of objects depending on the environment we are in.
- When we’re working with many small objects or components that share the same properties.
Say you are replicating methods several times. If you have a bug in the one you copy, all of them will have that bug, and all of them will need to be fixed one by one. If you use the factory pattern, you only need to fix the one bug!
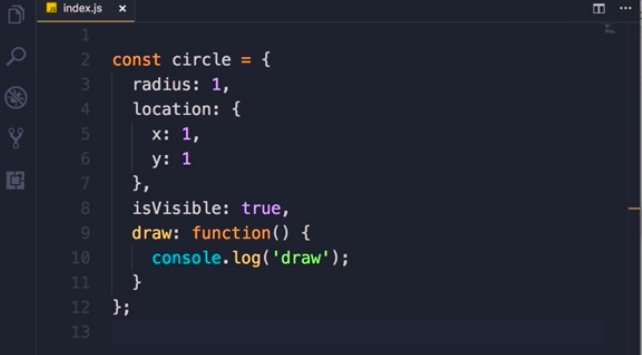
Here, if you wanted to create two circle objects, you’d have to replicate this exact code twice. Since everything is hard coded, there’s no flexibility. And if you have a bug, weeeell…
With the function createCircle, you can create different instances, with the ability to change the radius, and other parameters if you wished.
This pattern can save a lot of time, BUT if applied to the wrong type of problem, it can introduce an unnecessarily great deal of complexity to an application!
For more riveting reads:
The Comprehensive Guide to JavaScript Design Patterns
Short video on Factory Functions
Understand the Factory Design Pattern in plain javascript
JavaScript Factory Functions with ES6+